Table of Contents
Introduction
In this tutorial, How to run shell commands in Python. the ability to automate tasks and scripts is invaluable, and Python offers robust tools to execute these operations efficiently. this guide will provide the necessary insights and examples to integrate shell commands within your Python applications.
- Use subprocess module
- Use OS module
- Use sh library
Run shell commands in Python
Use subprocess module
You can use the subprocess module in Python to run shell commands. The subprocess.run() function can be used to run a command and return the output.
Here is an example of how to use the subprocess.run() function to run the ls command and print the output:
import subprocess
result = subprocess.run(['ls', '-l'], stdout=subprocess.PIPE)
print(result.stdout.decode())
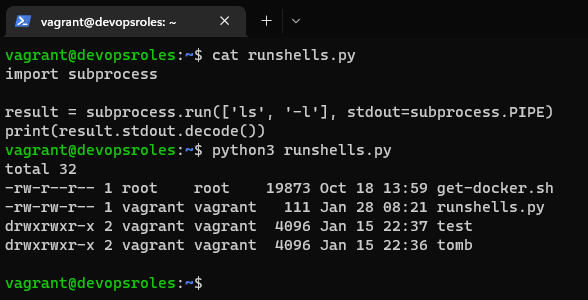
You can also use subprocess.Popen to run shell commands and access the input/output channels of the commands.
import subprocess
p = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = p.communicate()
print(stdout.decode())
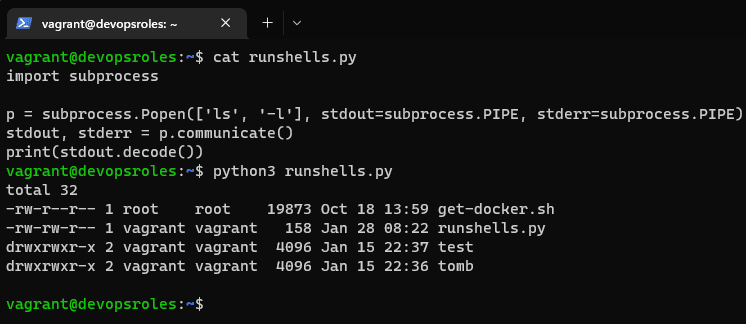
However, it’s generally recommended to avoid using shell commands in python, and instead use python libraries that provide equivalent functionality, as it is more secure and less error-prone.
use os module
The os module in Python provides a way to interact with the operating system and can be used to run shell commands as well.
Here is an example of how to use the os.system() function to run the ls command and print the output:
import os
os.system('ls -l')
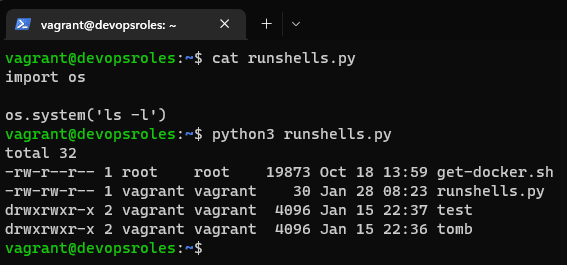
Alternatively, you can use the os.popen() function to run a command and return the output as a file object, which can be read using the read() or readlines() method.
import os
output = os.popen('ls -l').read()
print(output)
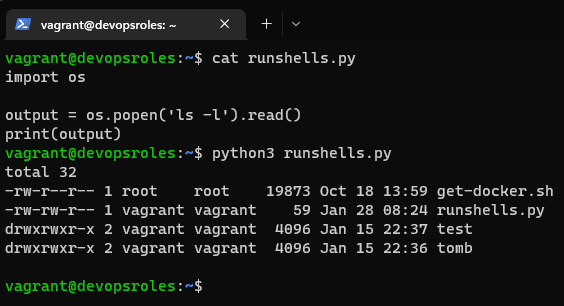
You can also use os.popen() and os.popen3() to run shell commands and access the input/output channels of the commands.
import os
p = os.popen3('ls -l')
stdout, stderr = p.communicate()
print(stdout)
It’s worth noting that the os.system() and os.popen() methods are considered old and are not recommended to use. subprocess module is recommended instead, as it provides more control over the process being executed and is considered more secure.
use sh library
The sh library is a Python library that provides a simple way to run shell commands, it’s a wrapper around the subprocess module, and it provides a more convenient interface for running shell commands and handling the output.
Here is an example of how to use the sh library to run the ls command and print the output:
from sh import ls
print(ls("-l"))
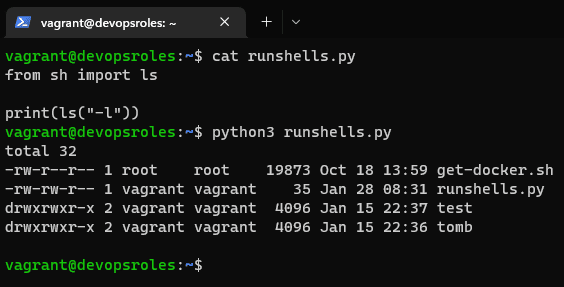
You can also use sh to run shell commands and access the input/output channels of the command.
from sh import ls
output = ls("-l", _iter=True)
for line in output:
print(line)
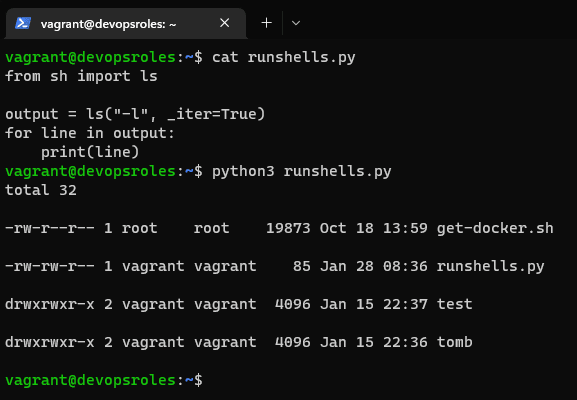
You can also capture the output of a command to a variable
from sh import ls
output = ls("-l", _ok_code=[0,1])
print(output)
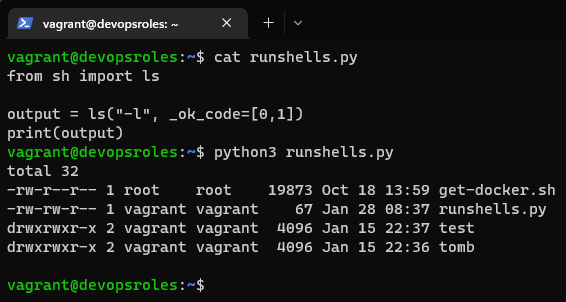
It’s worth noting that the sh library provides a very convenient way to run shell commands and handle the output, but it can be less secure, as it allows arbitrary command execution, so it’s recommended to use it with caution.
Conclusion
Throughout this tutorial, we explored various methods for executing shell commands using Python, focusing on the subprocess
module, os
module, and the sh
library. Each method offers unique advantages depending on your specific needs, from enhanced security and control to simplicity and convenience.
You have now learned how to run shell commands in Python. I hope you find this tutorial useful. Thank you for reading theΒ DevopsRolesΒ page!