Table of Contents
Introduction
How to Generate a Random Number in Python. Randomness plays a critical role in programming, enabling tasks ranging from data sampling to security.
In Python, the random
module offers versatile tools for generating random numbers and shuffling sequences, crucial for simulations, games, and more.
This article delves into six key functions of Python random
module, explaining their use and importance.
Random Number Generation in Python
What is the Random Module?
The Random Module is a built-in Python library. This means once you have Python installed on your computer, the Random Module is ready to use! It contains several functions to help you generate random numbers and perform actions on lists randomly. Let’s go through some of these functions:
1. seed() Function:
The seed()
function initializes the random number generator, allowing for the creation of reproducible sequences of random numbers. This is particularly useful for debugging or scientific research where repeatability is necessary.
Example:
import random
random.seed(10)
print(random.random())
Imagery: A flowchart beginning with setting a seed value, leading to a consistent random number sequence.
2. getstate() Function:
getstate()
captures the current state of the random number generator, enabling the preservation and replication of the sequence of random numbers.
Example:
import random
state = random.getstate()
print(random.random())
random.setstate(state)
print(random.random())
Imagery: A diagram showing the saving and restoring process of the generator’s state to reproduce a random number.
3. randrange() Function:
This function returns a randomly selected element from the specified range, exclusive of the endpoint. It’s useful for obtaining an integer within a range.
import random
print(random.randrange(1, 10))
Imagery: A number line from 1 to 10, with arrows indicating a range from 1 to 9.
4. randint() Function:
randint()
is similar to randrange()
, but inclusive of both endpoints, perfect for cases requiring a random integer within a fixed set of bounds.
Example:
import random
print(random.randint(1, 10))
Imagery: A number line from 1 to 10, including both endpoints, highlighting the function’s inclusivity.
5. choice() Function:
The choice()
function randomly selects and returns an element from a non-empty sequence, such as a list.
Example:
import random
items = ['apple', 'banana', 'cherry']
print(random.choice(items))
Imagery: Three fruits (apple, banana, cherry) with an arrow pointing randomly at one, illustrating the selection process.
6. shuffle() Function:
shuffle()
randomly reorders the elements in a list, commonly used for mixing or dealing cards in a game.
Example:
import random
cards = ['Ace', 'King', 'Queen', 'Jack']
random.shuffle(cards)
print(cards)
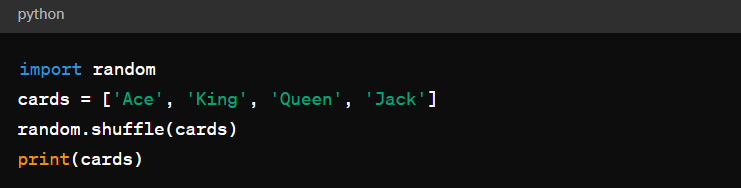
Imagery: A sequence of cards displayed before and after shuffling, demonstrating the randomization effect.
Conclusion
Mastering the random
module in Python empowers programmers to implement randomness in their projects effectively, whether for data analysis, gaming, or simulation. By understanding and utilizing these six functions, developers can enhance the unpredictability and variety in their programs, making them more dynamic and engaging. Thank you for reading the DevopsRoles page!