Table of Contents
Introduction: Harnessing the Power of Nested Lists in Terraform
Terraform, HashiCorp’s Infrastructure as Code (IaC) tool, empowers users to define and provision infrastructure through code. While Terraform excels at managing individual resources, the complexity of modern systems often demands the ability to handle nested structures and relationships. This is where the ability to build a list of lists with a Terraform for loop becomes crucial. This article provides a comprehensive guide to mastering this technique, equipping you with the knowledge to efficiently manage even the most intricate infrastructure deployments. Understanding how to build a list of lists with Terraform for loops is vital for DevOps engineers and system administrators who need to automate the provisioning of complex, interconnected resources.
Understanding Terraform Lists and For Loops
Before diving into nested lists, let’s establish a solid foundation in Terraform’s core concepts. A Terraform list is an ordered collection of elements. These elements can be any valid Terraform data type, including strings, numbers, maps, and even other lists. This allows for the creation of complex, hierarchical data structures. Terraform’s for
loop is a powerful construct used to iterate over lists and maps, generating multiple resources or configuring values based on the loop’s contents. Combining these two features enables the creation of dynamic, multi-dimensional structures like lists of lists.
Basic List Creation in Terraform
Let’s start with a simple example of creating a list in Terraform:
variable "my_list" {
type = list(string)
default = ["apple", "banana", "cherry"]
}
output "my_list_output" {
value = var.my_list
}
This code defines a variable my_list
containing a list of strings. The output
block then displays the contents of this list.
Introducing the Terraform for Loop
The for
loop allows iteration over lists. Here’s a basic example:
variable "my_list" {
type = list(string)
default = ["apple", "banana", "cherry"]
}
resource "null_resource" "example" {
count = length(var.my_list)
provisioner "local-exec" {
command = "echo ${var.my_list[count.index]}"
}
}
This creates a null_resource
for each element in my_list
, printing each element using a local-exec provisioner. The count.index
variable provides the index of the current element during iteration.
Building a List of Lists with Terraform For Loops
Now, let’s move on to the core topic: constructing a list of lists using Terraform’s for
loop. The key is to use nested loops, one for each level of the nested structure.
Example: A Simple List of Lists
Consider a scenario where you need to create a list of security groups, each containing a list of inbound rules.
variable "security_groups" {
type = list(object({
name = string
rules = list(object({
protocol = string
port = number
}))
}))
default = [
{
name = "web_servers"
rules = [
{ protocol = "tcp", port = 80 },
{ protocol = "tcp", port = 443 },
]
},
{
name = "database_servers"
rules = [
{ protocol = "tcp", port = 3306 },
]
},
]
}
resource "aws_security_group" "example" {
for_each = toset(var.security_groups)
name = each.value.name
description = "Security group for ${each.value.name}"
# ...rest of the aws_security_group configuration... This would require further definition based on your AWS infrastructure. This is just a simplified example.
}
#Example of how to access nested list inside a for loop (this would need more aws specific resource blocks to be fully functional)
resource "null_resource" "print_rules" {
for_each = {for k, v in var.security_groups : k => v}
provisioner "local-exec" {
command = "echo 'Security group ${each.value.name} has rules: ${jsonencode(each.value.rules)}'"
}
}
This example demonstrates the creation of a list of objects, where each object (representing a security group) contains a list of rules. Note the usage of for_each
which iterates over the list of security groups. While this doesn’t directly manipulate a list of lists in a nested loop, it shows how to utilize a list that inherently contains nested list structures. Accessing and manipulating this nested structure inside the loop using each.value.rules
is vital.
Advanced Scenario: Dynamic List Generation
Let’s create a more dynamic example, where the number of nested lists is determined by a variable:
variable "num_groups" {
type = number
default = 3
}
variable "rules_per_group" {
type = number
default = 2
}
locals {
groups = [for i in range(var.num_groups) : [for j in range(var.rules_per_group) : {port = i * var.rules_per_group + j + 8080}]]
}
output "groups" {
value = local.groups
}
#Further actions would be applied here based on your individual needs and infrastructure.
This code generates a list of lists dynamically. The outer loop creates num_groups
lists, and the inner loop populates each with rules_per_group
objects, each with a unique port number. This highlights the power of nested loops for creating complex, configurable structures.
Use Cases and Practical Applications
Building lists of lists with Terraform for loops has several practical applications:
- Network Configuration: Managing multiple subnets, each with its own set of security groups and associated rules.
- Database Deployment: Creating multiple databases, each with its own set of users and permissions.
- Application Deployment: Deploying multiple applications across different environments, each with its own configuration settings.
- Cloud Resource Management: Orchestrating the deployment and management of various cloud resources, such as virtual machines, load balancers, and storage.
FAQ Section
Q1: Can I use nested for
loops with other Terraform constructs like count
?
A1: Yes, you can combine nested for
loops with count
, for_each
, and other Terraform constructs. However, careful planning is essential to avoid unexpected behavior or conflicts. Understanding the order of evaluation is crucial for correct functionality.
Q2: How can I debug issues when working with nested lists in Terraform?
A2: Terraform’s output
block is invaluable for debugging. Print out intermediate values from your loops to inspect the structure and contents of your lists at various stages of execution. Also, the terraform console
command allows interactive inspection of your Terraform state.
Q3: What are the limitations of using nested loops for very large datasets?
A3: For extremely large datasets, nested loops can become computationally expensive. Consider alternative approaches, such as data transformations using external tools or leveraging Terraform’s data sources for pre-processed data.
Q4: Are there alternative approaches to building complex nested structures besides nested for loops?
A4: Yes, you can utilize Terraform’s data sources to fetch pre-structured data from external sources (e.g., CSV files, APIs). This can streamline the process, especially for complex configurations.
Q5: How can I handle errors gracefully when working with nested loops in Terraform?
A5: Employ proper error handling using try
and catch
blocks to gracefully manage exceptions that might occur during the loop’s execution.
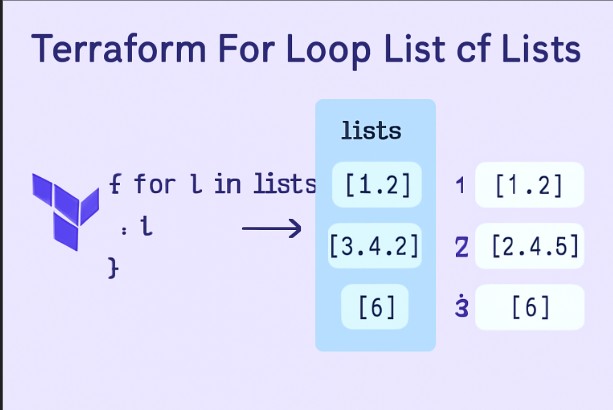
Conclusion Terraform For Loop List of Lists
Building a list of lists with Terraform for loops is a powerful technique for managing complex infrastructure. This method provides flexibility and scalability, enabling you to efficiently define and provision intricate systems. By understanding the fundamentals of Terraform lists, for
loops, and employing best practices for error handling and debugging, you can effectively leverage this technique to create robust and maintainable infrastructure code. Remember to carefully plan your code structure and leverage Terraform’s debugging capabilities to avoid common pitfalls when dealing with nested data structures. Proper use of this approach will lead to more efficient and reliable infrastructure management. Thank you for reading theΒ DevopsRolesΒ page!